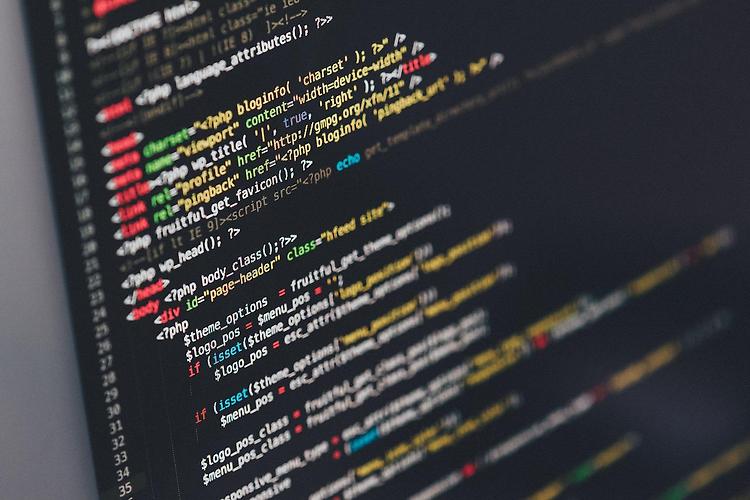
문제 지훈이가 불에 타기 전에 미로를 탈출할 수 있으면 탈출 시간을 출력하고 불가능하다면 IMPOSSIBE을 출력하는 문제로 불은 매시간 상하좌우 4방향으로 한 칸씩 확산되고 지훈이는 상하좌우 한 칸씩 이동할 수 있다. (지훈이와 불은 모두 벽을 통과할 수는 없다) 접근 방식 지훈이와 불을 동시에 출발시켜서 지훈이가 미로를 탈출하는지를 확인하면 되는 문제로 접근 방식을 떠올리는 건 간단한 문제다. 이제 어떻게 동시에 출발 시키냐는건데 일단 반복문에서 동시에 불과 지훈이를 한 번에 같이 탐색하는 건 불가능하다. (추후에 백트래킹을 배우면 가능하다.) 그래서 불과 지훈이를 각각 따로 움직여서 기록을 남길 건데, 이전에 풀어왔던 BFS 문제들처럼 거리를 남겨주면 된다. 불들의 거리를 모두 남긴 이후에 지훈이를..